Tic-tac-toe AI
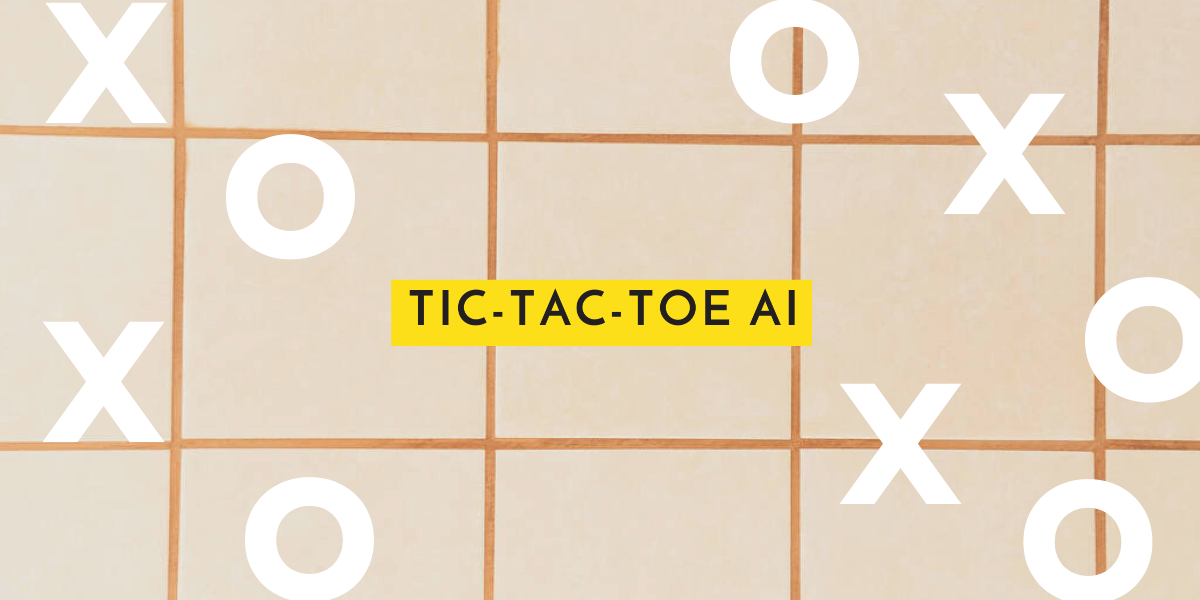
In this project, I created a tic-tac-toe AI based on the minimax algorithm to play against.
I used Python 3.9 for this project, with no other external dependencies.
Motivation
I wanted to practise my object-oriented programming (OOP) concepts, and also try out the minimax algorithm to make an AI player for the user to play against. I chose tic-tac-toe as it is a simple two-player game with a maximum of 9 moves.
Approach
I defined the TicTacToe board and Player as classes and gave them their own methods. Thereafter a Human class, a RandomComputer class, and a MinimaxComputer class are created as child classes of the Player class. Three python files were created to run the game, to hold the board class, and the different player classes.
Initially, I created the game logic with the game in its simplest form, with two human players. I also chose to represent the TicTacToe board using a list of 9 elements, rather than a list of lists, for simplicity.
Once the game is up, I created a simple AI player that randomly chooses a valid move for each turn.
Finally, I created an AI that uses the minimax algorithm. The minimax algorithm works by assigning a utility score to each potential move of a board state, and the algorithm is called recursively and swapping players repeatedly to simulate the entire game. After evaluating all potential moves of a given board state, the move with the highest utility score (the best move) is chosen.
Improvements
This project can be extended by adding an AI using reinforcement learning (RL), i.e. learning the game by playing itself a large number of times. It will be interesting to see the results of a head-to-head battle between the MinimaxComputer and the RLComputer.
Takeaways
Through this project, I became more familiar with writing code using the OOP approach rather than defining many functions in a single file. Writing code in this way not only makes it easier to add documentation, but also modularizes the codebase so that it is easier to add new features in the future (e.g. an AI using reinforcement learning).
While debugging, I also tried the logging library as well as using breakpoints and VSCode built-in debug features.
Also, I got to work with git and GitHub, which is an indispensable skill as a data scientist and developer.